《Build a Large Language Model (From Scratch)》
- Author: Sebastian Raschka
- BOOK: Build a Large Language Model (From Scratch)
- GitHub: rasbt/LLMs-from-scratch
- 中英文pdf版本, 可联系我获取
- 如有侵权,请联系删除
Setup
参考
setup/01_optional-python-setup-preferences
.setup/02_installing-python-libraries
按照步骤配置环境:
1 | git clone --depth 1 https://github.com/rasbt/LLMs-from-scratch.git |
在这里遇到了以下问题:
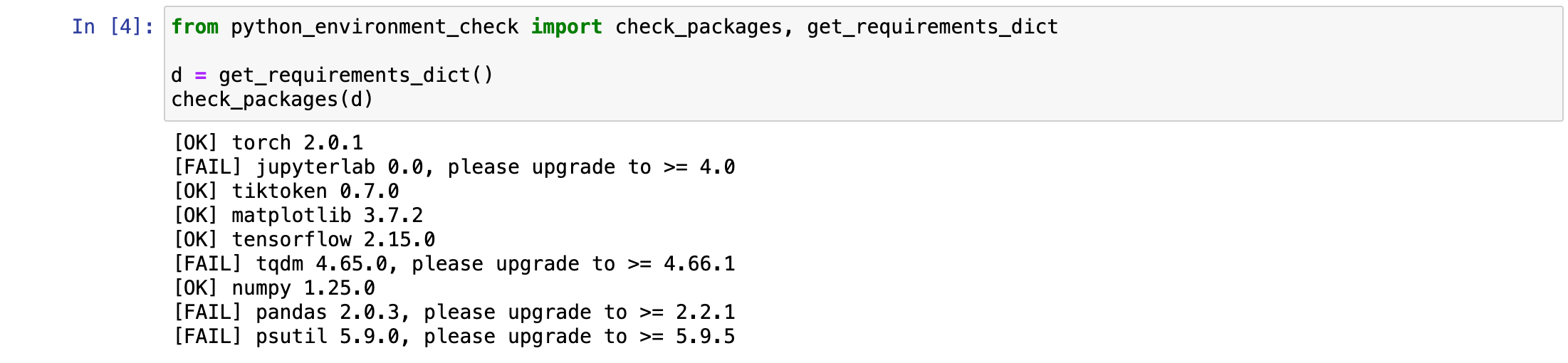
解决方案:
1 | hash -r |
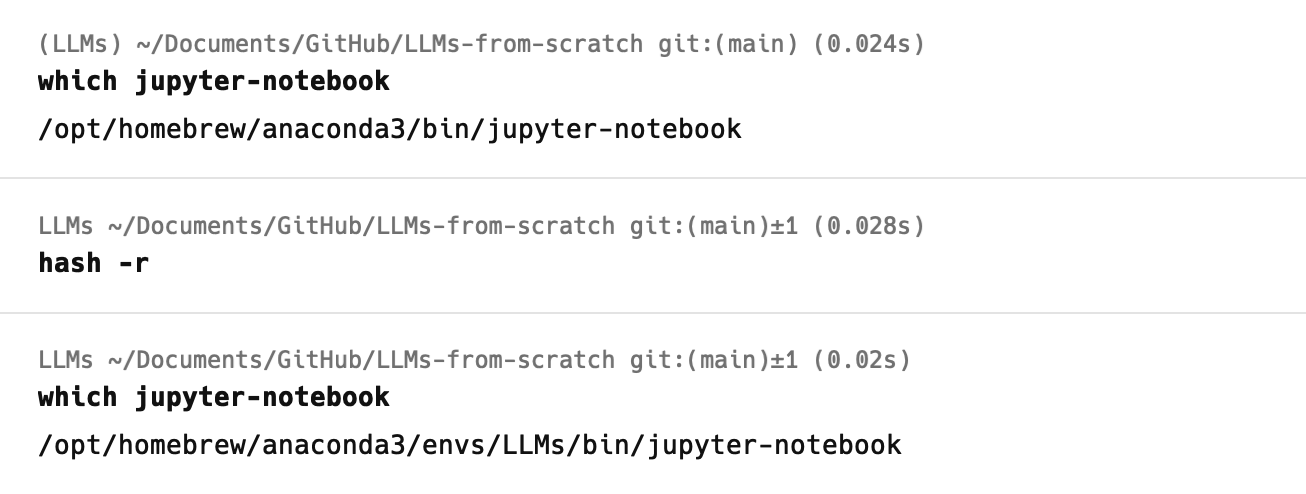
解释:
hash
是一个 Bash 内建命令,用于查找并记住命令的位置。如果你在安装了新的软件之后,想要立即使用它,但是 Bash 仍然使用旧的命令,那么你可以使用hash -r
命令来刷新 Bash 的命令缓存。